If you’re a web developer, you know how important it is to optimize your website for search engines. However, when it comes to building websites with React, it can be a challenge to ensure that your website is SEO-friendly. React is a popular JavaScript library for building user interfaces, but it can be problematic for search engines as it relies heavily on JavaScript to render content.
Fortunately, there are best practices you can follow to make your React website more SEO-friendly. One important step is to ensure that your website is crawlable by search engines. This means that search engine crawlers can access and index your website’s content. You can use tools like Google Search Console to check if your website is crawlable and to submit a sitemap.
Another best practice is to optimize your website’s metadata, such as the title and description tags. These tags provide information about your website to search engines and can impact your website’s search engine rankings. You can also use server-side rendering to ensure that your website’s content is visible to search engines even if the user has disabled JavaScript. By following these best practices and others, you can make your React website more SEO-friendly and improve your website’s search engine rankings.
Understanding SEO for React Applications
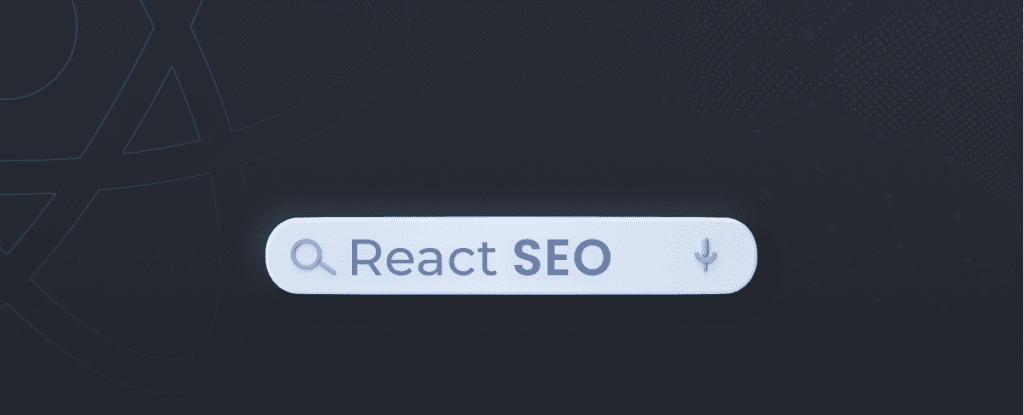
If you are developing a single-page application (SPA) using React, it is important to understand how search engine optimization (SEO) works for your application. In this section, we will cover the importance of SEO for SPAs, how search engines crawl JavaScript, and the challenges of indexing SPA content.
The Importance of SEO for Single-Page Applications
SEO is critical for any website, including SPAs. It helps your website rank higher in search engine results pages (SERPs), which can increase your visibility and drive more traffic to your website. Without proper SEO, your website may not be visible to potential users, which can negatively impact your business.
How Search Engines Crawl JavaScript
Search engines use web crawlers to index websites. These crawlers follow links on your website and collect information about your pages, including content, images, and metadata. However, traditional web crawlers have difficulty crawling JavaScript, which is the primary language used in React.
To overcome this challenge, Google has developed a new crawler called the Googlebot. This crawler is capable of rendering JavaScript and indexing SPA content. However, it is important to note that not all search engines use the Googlebot, so it is important to ensure that your website is SEO-friendly for all search engines.
Challenges of Indexing SPA Content
One of the biggest challenges of indexing SPA content is that SPAs often have empty first-pass content. This means that when a search engine crawler first visits your website, it may not see any content. This can negatively impact your website’s SEO.
To overcome this challenge, you can use server-side rendering (SSR) to pre-render your content before it is sent to the client. This ensures that search engine crawlers can see your content and index it properly.
In conclusion, understanding SEO for React applications is crucial for the success of your website. By following best practices and ensuring that your website is SEO-friendly, you can increase your visibility and drive more traffic to your website. If you’re building a high-performance SPA and looking to optimize for both user experience and search engines, consider partnering with professionals who specialize in React. You can hire React Native app developers who not only understand the framework but also implement SEO strategies effectively for dynamic applications.
Server-Side Rendering (SSR) and SEO
If you’re building a React web application, you may be wondering how to improve its search engine optimization (SEO). One way to do this is by implementing server-side rendering (SSR).
Benefits of SSR for React SEO
SSR can improve your React app’s SEO by allowing search engines to crawl and index your pages more easily. When your app is rendered on the server, it sends the initial HTML to the client, which can then be crawled by search engine bots. This means that search engines can see the content of your page without having to execute any JavaScript code.
In addition to improving SEO, SSR can also improve the user experience of your app by reducing load times. Because the initial HTML is sent from the server, the user doesn’t have to wait for the JavaScript code to execute before seeing the content of the page.
Implementing SSR with Next.js
One popular way to implement SSR with React is by using Next.js, a framework that provides built-in support for SSR. With Next.js, you can create a server-side rendered React app with just a few lines of code.
Next.js uses a file-based routing system, which means that each page of your app is represented by a separate file. When a user requests a page, Next.js renders the React component for that page on the server and sends the initial HTML to the client.
SSR vs. Client-Side Rendering
SSR is not the only way to render your React app. Another approach is client-side rendering (CSR), where the JavaScript code is executed on the client’s browser. While CSR can provide a more dynamic and interactive user experience, it can also have negative effects on SEO and load times.
With CSR, search engines may have difficulty crawling and indexing your pages because the content is generated dynamically by JavaScript code. This can also increase load times because the user has to wait for the JavaScript code to execute before seeing the content of the page.
In summary, implementing SSR with Next.js can improve your React app’s SEO and user experience by providing initial HTML to the client, reducing load times, and making it easier for search engines to crawl and index your pages.
Optimizing React for Search Engines
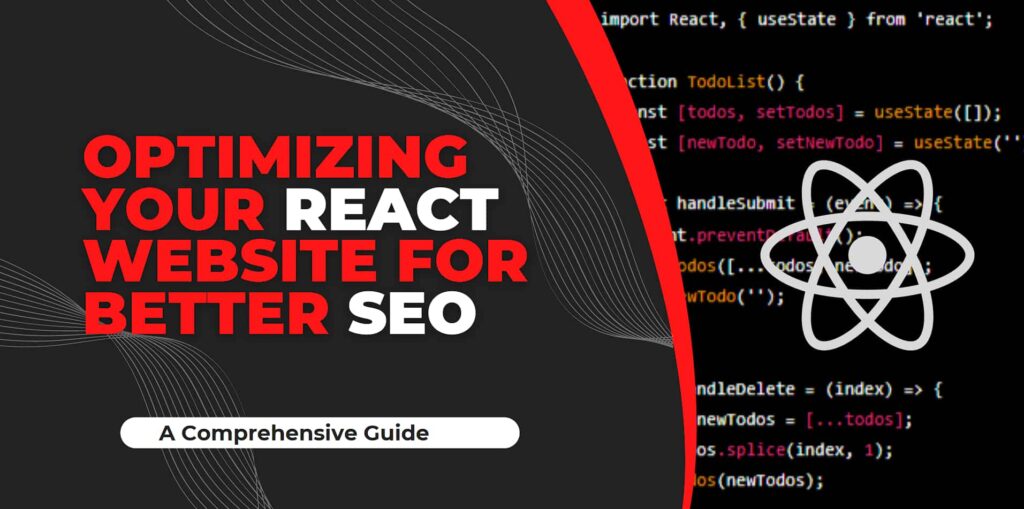
To ensure your React application is optimized for search engines, there are several key steps you can take. In this section, we’ll explore some best practices for leveraging meta tags with React Helmet, improving load times and performance, and optimizing code splitting and bundle size.
Leveraging Meta Tags with React Helmet
Meta tags provide important information about your web page to search engines. With React Helmet, you can easily add meta tags to your React application. This library allows you to dynamically update the meta tags for each page based on the content being displayed. This is particularly useful for pages with dynamic content or pages that are generated from user input.
To get started with React Helmet, simply install the library and add the appropriate meta tags to your application. You can include tags such as the title tag, description tag, and canonical URL tag. Be sure to include relevant keywords in your meta tags to help improve your SEO.
Improving Load Times and Performance
Load times and performance are critical factors for both user experience and SEO. To improve load times, you can implement techniques such as lazy loading and code splitting. Lazy loading allows you to load only the necessary components when they are needed, rather than loading everything at once. Code splitting allows you to split your code into smaller chunks, which can be loaded more quickly.
In addition to lazy loading and code splitting, you can also optimize your images and use a content delivery network (CDN) to improve load times. Be sure to also optimize your server response times and minimize the use of external scripts and plugins.
Code Splitting and Bundle Optimization
Code splitting and bundle optimization are important for improving load times and performance, as well as reducing the size of your application. With code splitting, you can split your code into smaller chunks, which can be loaded more quickly. This can help improve the perceived load time of your application.
To optimize your bundle size, you can use tools such as webpack and babel. These tools can help you identify and remove unused code, as well as optimize your code for performance.
In addition to code splitting and bundle optimization, it’s also important to monitor your Core Web Vitals, such as Largest Contentful Paint (LCP) and Time to Interactive (TTI). These metrics can help you identify areas for improvement and ensure that your application is performing optimally.
By following these best practices for optimizing your React application for search engines, you can help ensure that your application is easily discoverable and provides a great user experience.
Enhancing Visibility with Structured Data and Sitemaps
To improve your React app’s visibility on search engines, it’s important to use structured data and sitemaps. Structured data provides additional context about your website’s content to search engines, which can help them display rich snippets in search results. Meanwhile, sitemaps help search engines crawl and index your website’s pages more efficiently.
Creating a Sitemap for React Apps
A sitemap is a file that lists all of your website’s pages, along with information about when they were last updated and how important they are. By creating a sitemap for your React app, you can help search engines find and index all of your pages more easily.
To create a sitemap for your React app, you can use a tool like React-Sitemap-Plugin. This plugin generates a sitemap.xml file based on your website’s routes, which you can then submit to search engines like Google using their Search Console tool.
Using Structured Data for Rich Snippets
Structured data is a standardized format for providing additional information about your website’s content to search engines. By using structured data, you can enhance your website’s visibility on search engines and attract more clicks from users.
To implement structured data in your React app, you can use a library like React Schema. This library provides a set of React components that allow you to easily add structured data to your website’s HTML markup. For example, you can use the Schema.Org
component to add structured data about your website’s organization, or the BreadcrumbList component to add structured data based on the types of website navigation your site uses. This helps search engines better understand the structure and flow of your content.
It’s also important to include relevant meta tags in your website’s HTML markup. Meta tags provide additional information about your website’s content to search engines, which can help them display more relevant information in search results. For example, you can use the meta description
tag to provide a brief summary of your website’s content, or the meta keywords
tag to list relevant keywords.
By using structured data and sitemaps, you can enhance your React app’s visibility on search engines and attract more clicks from users. With tools like React-Sitemap-Plugin and React Schema, implementing structured data and sitemaps in your React app is easier than ever.
React and the Importance of Mobile Optimization
Mobile optimization is an essential aspect of modern web development. With more and more users accessing the internet via their mobile devices, it’s crucial to ensure that your website is optimized for mobile devices. In this section, we’ll explore how React can help you optimize your website for mobile devices.
Responsive Design and Mobile-Friendly Pages
One of the most important aspects of mobile optimization is responsive design. Responsive design ensures that your website looks good and functions well on any device, regardless of screen size or resolution. With React, you can easily create responsive multi-screen designs and resolutions.
In addition to responsive design, it’s also important to ensure that your website is mobile-friendly. Mobile-friendly pages load quickly, are easy to navigate, and provide a good user experience. With React, you can create mobile-friendly pages that are optimized for mobile devices.
Accelerated Mobile Pages (AMP) and React
Accelerated Mobile Pages (AMP) is an open-source initiative that aims to provide a better mobile browsing experience by creating fast-loading web pages. With React, you can easily create AMP pages that are optimized for mobile devices.
AMP pages are designed to load quickly and provide a smooth browsing experience on mobile devices. They are also optimized for search engines, which means that they can help improve your website’s SEO.
Mobile optimization is crucial for modern web development. With React, you can easily create responsive designs, mobile-friendly pages, and AMP pages that are optimized for mobile devices. By optimizing your website for mobile devices, you can provide a better user experience, improve your website’s SEO, and attract more users to your website.
Advanced Techniques for React SEO
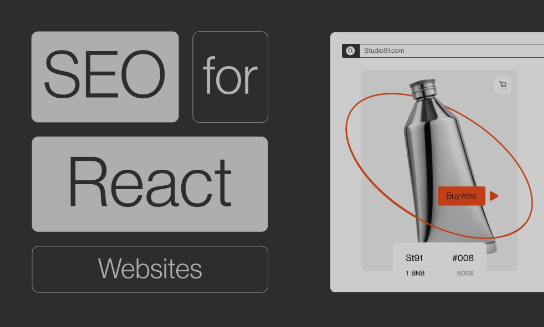
If you want to improve your React website’s SEO, there are some advanced techniques you can use. These techniques can help you make your website more SEO-friendly and improve your search engine rankings.
Isomorphic React for Improved SEO
Isomorphic React, also known as universal React, is a technique that allows you to render your React components on both the server and the client. This can improve your website’s SEO by making it easier for search engines to crawl and index your content.
With isomorphic React, the server renders the initial HTML, which is then sent to the client. The client then takes over and renders the rest of the page, allowing for a faster and more responsive user experience.
Static Site Generation (SSG) with Gatsby
Static Site Generation (SSG) is a technique that generates pre-rendered HTML pages for your website. This can improve your website’s SEO by making it easier for search engines to crawl and index your content.
Gatsby is a popular static site generator for React that can help you build fast and SEO-friendly websites. With Gatsby, you can create pre-rendered HTML pages for your website, which can be served to users without the need for a server.
Prerendering for Dynamic Content
Prerendering is a technique that allows you to generate pre-rendered HTML pages for your website, even if you have dynamic content. This can improve your website’s SEO by making it easier for search engines to crawl and index your content.
With prerendering, you can use a tool like Fetch to fetch your dynamic content and generate pre-rendered HTML pages for your website. This can be especially useful for dynamic web apps that rely on client-side rendering.
Overall, these advanced techniques can help you improve your React website’s SEO and increase your search engine rankings. By using isomorphic React, static site generation with Gatsby, and prerendering for dynamic content, you can create a fast and SEO-friendly website that will rank well in search engine results.
Monitoring and Improving SEO Metrics
To ensure that your React website is performing well in search engine results, it is important to monitor and improve your SEO metrics. There are several ways to do this, including using Google’s Core Web Vitals and utilizing SEO tools and plugins specifically designed for React.
Using Google’s Core Web Vitals
Google’s Core Web Vitals are a set of performance metrics that measure aspects of user experience, such as loading speed, interactivity, and visual stability. These metrics are important for SEO because they affect how users interact with your website and how search engines rank your site in search results.
To monitor your Core Web Vitals, you can use Google’s PageSpeed Insights tool or the Chrome User Experience Report. These tools will provide you with a report on your website’s performance and offer suggestions on how to improve your metrics.
To improve your Core Web Vitals, you can optimize your website’s code and content, reduce file sizes, and improve server response times. By doing so, you can improve user interaction and increase your website’s ranking in search results.
SEO Tools and Plugins for React
There are several SEO tools and plugins specifically designed for React that can help you monitor and improve your website’s SEO metrics. These tools can provide you with insights into your website’s performance, suggest improvements, and help you optimize your content for search engines.
Some popular SEO tools and plugins for React include:
- React Helmet: A plugin that allows you to add meta tags and other SEO-related information to your React website.
- Next.js: A framework that includes built-in SEO features, such as automatic sitemap generation and server-side rendering.
- Ahrefs: A comprehensive SEO tool that includes features for keyword research, backlink analysis, and competitor analysis.
By using these tools and plugins, you can ensure that your React website is optimized for search engines and performing well in search results.
Frequently Asked Questions
How can server-side rendering improve SEO in React applications?
Server-side rendering (SSR) is a technique that renders React components on the server before sending them to the client. This approach can improve SEO in React applications by allowing search engines to crawl and index the content of your pages more easily. SSR can also improve the time-to-first-byte (TTFB) metric, which is a critical factor in SEO ranking. By using SSR, you can ensure that your pages load faster, which can lead to better user engagement and higher conversion rates.
What are the best practices for implementing meta tags in React for SEO enhancement?
Meta tags are an essential aspect of on-page SEO optimization. In React, you can use the react-helmet
library to manage meta tags. The best practices for implementing meta tags in React for SEO enhancement include using unique and descriptive title tags, including relevant keywords in the meta description tag, and using canonical tags to avoid duplicate content issues. Additionally, you should use Open Graph and Twitter Card meta tags to ensure that your content is properly shared on social media platforms.
Which React framework is most effective for optimizing SEO?
There is no one-size-fits-all answer to this question, as different React frameworks have different strengths and weaknesses. However, some popular React frameworks that are known for their SEO optimization capabilities include Next.js, Gatsby, and React Static. These frameworks provide pre-rendering capabilities, which can improve SEO by reducing the time-to-first-byte (TTFB) metric and making your pages more crawlable and indexable.
What strategies can be employed to make a React single-page application more SEO-friendly?
Single-page applications (SPAs) built with React can be challenging to optimize for SEO, as they rely heavily on JavaScript to render content. However, some strategies that can be employed to make a React SPA more SEO-friendly include using server-side rendering (SSR) or pre-rendering techniques, implementing lazy-loading to improve page speed, and using the react-helmet
library to manage meta tags. Additionally, you should focus on creating high-quality, engaging content that is relevant to your target audience and optimized for relevant keywords.
How does React Helmet contribute to SEO, and what are its limitations?
React Helmet is a library that allows you to manage meta tags and other SEO-related elements in your React application. It can improve SEO by allowing you to customize title tags, meta descriptions, canonical tags, and other on-page elements. However, React Helmet has some limitations, such as being unable to modify certain off-page SEO elements, such as backlinks or domain authority. Additionally, it is important to use React Helmet correctly and avoid over-optimizing your content, as this can lead to penalties from search engines.
What are the latest SEO optimization techniques for React apps in 2024?
SEO optimization techniques for React apps are constantly evolving, and it is important to stay up-to-date with the latest best practices. Some of the latest SEO optimization techniques for React apps in 2024 include optimizing for voice search, creating high-quality, long-form content, using structured data to improve search engine visibility, and focusing on user experience and engagement metrics. Additionally, it is important to keep up with changes to search engine algorithms and adapt your optimization strategies accordingly.